

Unlocking Database Power: A Comprehensive Guide to JDBC in Java
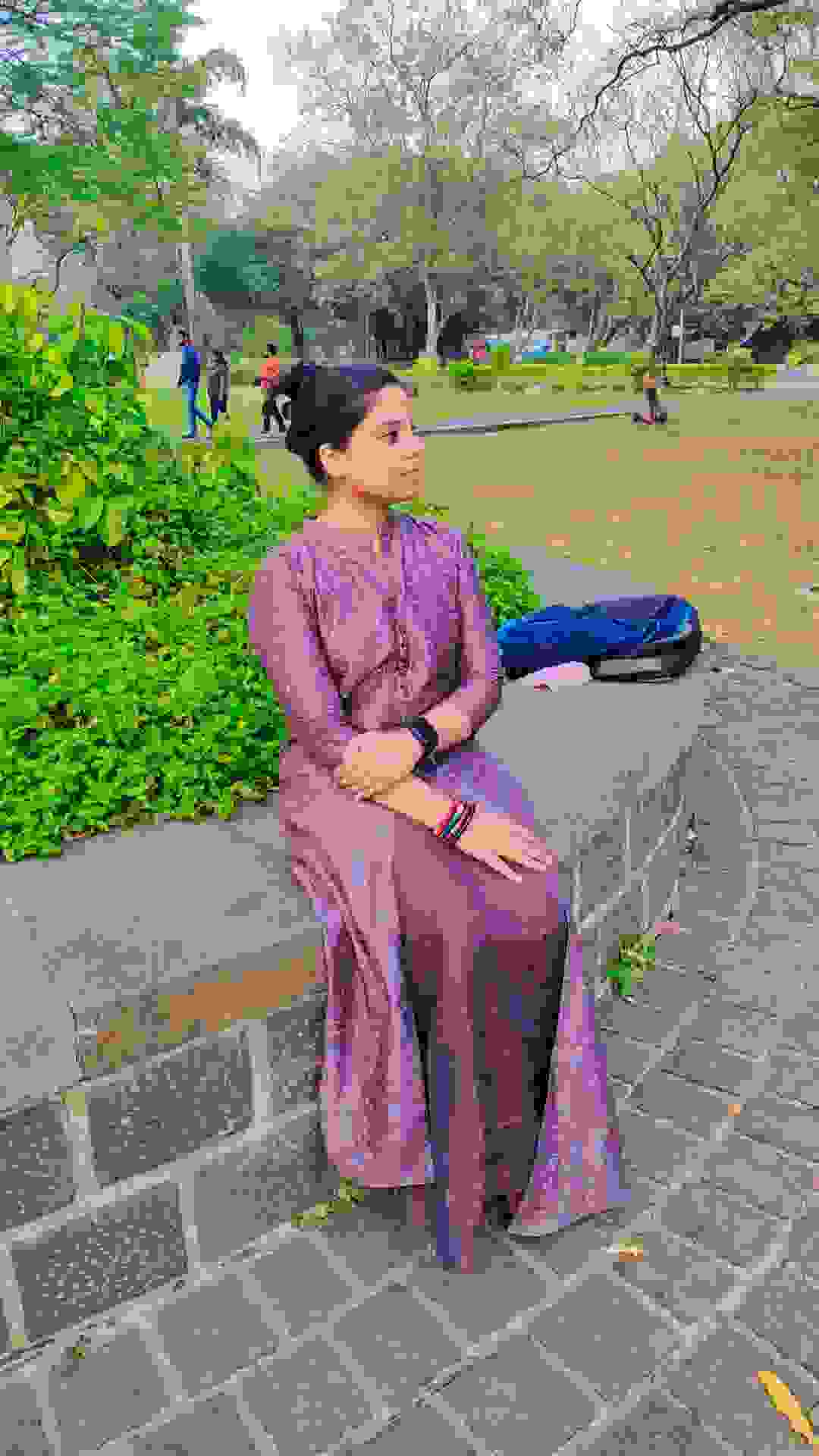
In this blog, weโre going to take a look at JDBC (Java Database Connectivity) which is an API for connecting and executing queries on a database.
Understanding the JDBC:
JDBC serves as a bridge between Java applications and databases, allowing seamless communication. It provides a standardized interface for Java developers to interact with various database management systems (DBMS) like MySQL, PostgreSQL, Oracle, and more.
JDBC (Java Database Connectivity) is a java API for connecting and interacting with database.
JDBC Drivers are software components that provide necessary functionality to connect java applications to connect different types of Databases.
when we are using JDBC there are several key Components like below and we briefly know about the component:
1.DriverManager: DriverManager is a Class .It manages a list of Database drivers. It helps establish a connection with the appropriate driver based on the database URL provided.
2.Connection: Connection is an Interface. It represents a connection with a specific database. It allows executing SQL statements and managing transactions.
3.Statements: The Statements is an Interface. It perform run or execute SQL queries against the database. There are different types of statements available, such as Statement, PreparedStatement, and CallableStatement, catering to various needs.
4.ResultSet: The ResultSet is an Interface. It represents the result set of a database query.
Basic Program flow or Workflow of JDBC:
Note: Make sure in your Machine installed one database like PostgreSQL, MySQL etc.. |
Here we know about ...
Note: Connection strings are used to establish connections to Databases. without connection string you can't connect to your database. |
CONNECTION STRING AND IT'S TYPE:
The connection string is used to connect from the java application to the specified database server. We need database hostname, database name, database username, database user password, and database port number to connect the database server using the connection string. We need a database driver to connect the specified database.
Type 1: Simple Connection String: This is a basic connection string format that typically includes the database URL, username, and password.
Example:
Type 2: Connection String with Properties: Some databases allow additional properties to be specified in the connection string, such as connection timeout, SSL settings, etc. These properties are appended to the URL.
Example:
Type 3: Connection String with connection Pooling: The connection string might include parameters related to connection pooling configuration, such as maximum pool size, idle timeout, etc.
Example:
Type 4: URL less connection String: Some JDBC drivers allow connections to be established without specifying a URL. Instead, you provide the necessary connection details programmatically.
Example:
Type 5: URL less connection String: Instead of directly specifying the connection details in the URL, you can use a DataSource object to manage connections. In this case, the connection string might just specify the JNDI (Java Naming and Directory Interface) name of the DataSource.
Example:
" When we Establish a Connection the code remain same for all connection string:"
DRIVERMANAGER AND IT'S USE:
The DriverManager is a class in Java is part of the JDBC (Java Database Connectivity) API.It manages a list of database drivers. The DriverManager class helps in loading the appropriate driver when a connection to a database is requested.
some important methods in the DriverManager class:
1.DriverManager. You typically don't need to call this method explicitly, as the drivers are automatically registered when their class is loaded.
2.getConnection(String url, String user, String password): This method attempts to establish a connection to the database specified by the given URL. It returns a Connection object representing the connection to the database.
3.getDrivers(): This method returns an Enumeration of all the currently loaded JDBC drivers.
Note: Above Establish connection is the example of DriverManager. |
STATEMENTS:
A statement is an interface used to execute SQL queries against a database.
There are three main types of statements in JDBC:
1.Statement:
A) Statement interface is typically used to execute simple SQL queries.
B) It's created by calling createStatement() method on a Connection object.
C) It executes SQL queries using executeQuery() method for SELECT statements,
and executeUpdate() method for INSERT, UPDATE,DELETE.
2.PreparedStatement:
A) PreparedStatement interface is used when you need to execute the same SQL statement multiple times with different parameter values.
B) It's created by calling prepareStatement() method on a Connection object with a parameterized SQL query.
C) It's precompiled, which makes it more efficient for repeated executions.
3.CallableStatement:
A) CallableStatement interface is used to execute stored procedures, functions, or anonymous PL/SQL blocks.
B) It's created by calling prepareCall() method on a Connection object with a callable SQL statement.
C) It allows passing input and output parameters to the stored procedure or function.
RESULTSET :
In JDBC (Java Database Connectivity), the ResultSet interface represents the result set of a database query.
A) Retrieving Data: The primary purpose of ResultSet is to provide access to the rows of data returned by a query. You can retrieve data from the result set using methods like getInt, getString, getDouble, etc., corresponding to the data types of the columns in the result set.
B) Traversing Rows: ResultSet maintains a cursor that points to the current row of data. You can move the cursor forward using methods like next(), previous(), first(), last(), absolute(int row), relative(int rows), etc., to navigate through the rows of the result set.
C) Retrieving Metadata: ResultSet provides methods to retrieve metadata about the columns in the result set, such as the column names, data types, and properties.
D) Updating Data: Depending on the type of ResultSet (either TYPE_SCROLL_INSENSITIVE, TYPE_SCROLL_SENSITIVE, or TYPE_FORWARD_ONLY), you may or may not be able to update the data in the result set and reflect those changes back to the database.
NOTE: when we retrive data from table then that time we use ResultSet. |
Git Repository: https://github.com/PallaviGajananGaikwad/JDBCDemo.git