

Exploring Random Numbers in Java
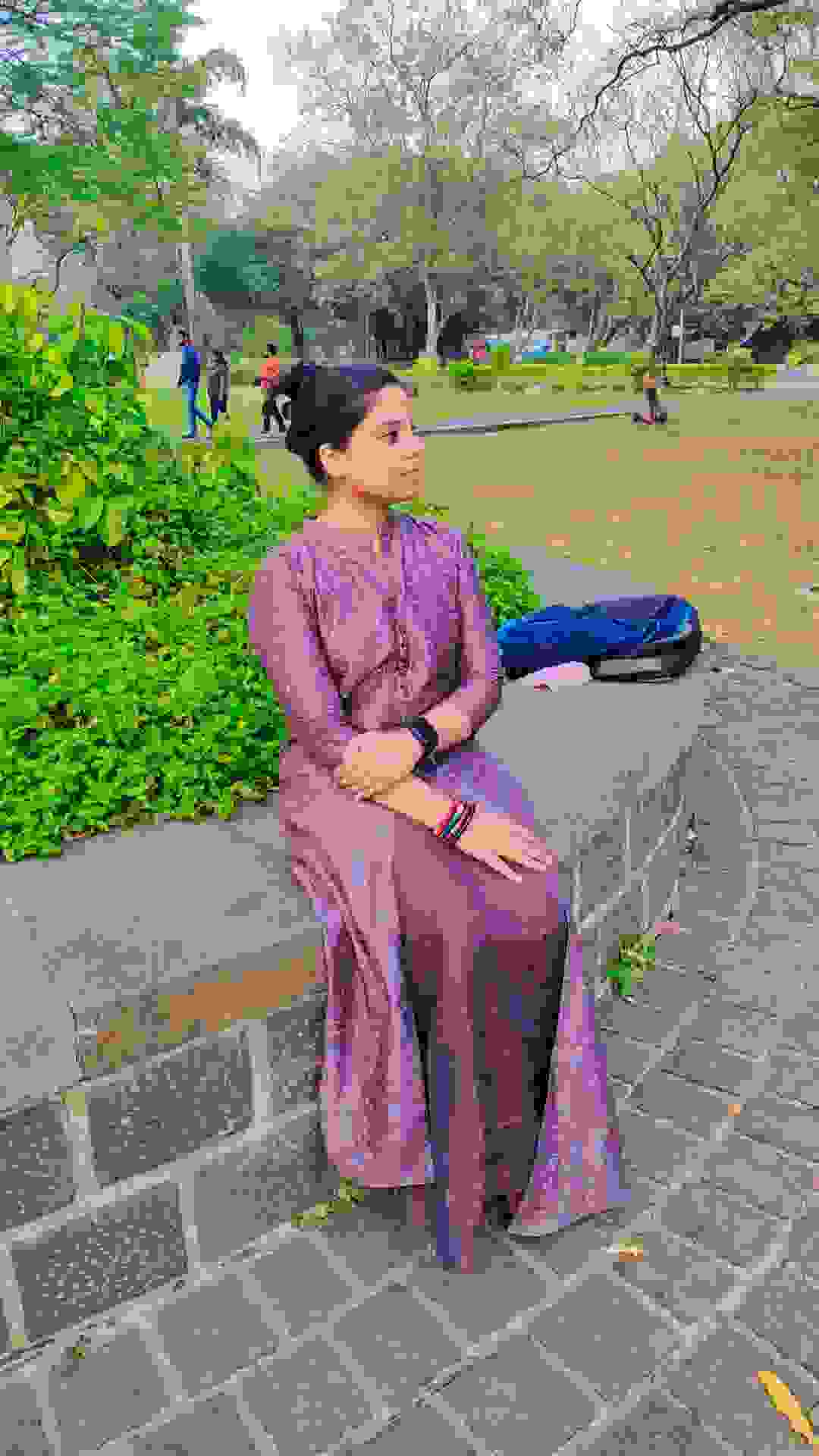
This Blog will introduce you to Random Number In Java and follow it up with a programmatic demonstration.
Following pointers will be covered in this blog,
1.Java.util.Random
2.Math.random()
3.Java.util.concurrent.ThreadLocalRandom class
There are three methods to generate Random numbers in java using built-in methods and classes.
Java.util.Random class
Math. random method
ThreadLocalRandom class
Let's see ...
Generating Random Integers
Approach 1:
Output:
Note: By using random class will get the any random value between 0 to 99 after executing the code means same value may or may not generate for next execution. |
Approach 2: Math. random method
The Math.random() method in Java is used to generate a random double value between 0.0 (inclusive) and 1.0 (exclusive). It returns a pseudo-random double value greater than or equal to 0.0 and less than 1.0.
Output:
Approach 3: Java.util.concurrent.ThreadLocalRandom class
A java.util.concurrent.ThreadLocalRandom is a utility class is useful when multiple threads .It improves performance and have less contention than Math.random() method.
Output:
GitRepository:https://github.com/PallaviGajananGaikwad/RandomNumbers.git
Conclusion:
Random number generation is a fundamental aspect of many Java applications. By leveraging the functionalities provided by java.util.Random, developers can introduce controlled randomness into their programs, enabling a wide range of functionalities from game development to statistical analysis.