

Returning Multiple Values from Functions: A Comparison of Java, Python, and GoLang
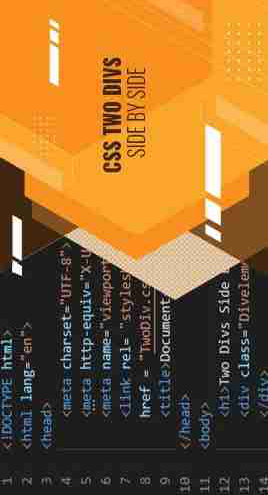
Functions are the building blocks of programming. They provide modularity and enable reusability. However, one common challenge across languages is how to return multiple values from a function efficiently and intuitively.
In this blog, we’ll explore how three popular programming languages—Java, Python, and GoLang—handle this scenario, with practical examples for each.
Lets understand this difference with a problem statement
You’re building a calculateInterest function that takes three parameters—principalAmount, RateOfInterest, and time—and needs to return both:
1 - Total Amount After Interest
2 - Interest Amount
The JAVA Way
Java, a statically typed, object-oriented language, doesn’t directly support multiple return values. Instead, developers often rely on collections, custom objects, or built-in classes like Map
or Pair
.
Key Takeaways for Java
1 - Use collections or custom objects to bundle multiple return values.
2 - While verbose, this approach is flexible for larger, complex datasets.
The PYTHON Way
Python, being a dynamically typed language, makes it incredibly easy to return multiple values using tuples. This is one of the language’s strong suits, ensuring clean and concise code.
Key Takeaways for Python:
1 - Python’s tuples make multiple return values intuitive and readable. (Note: When you use a return
statement with multiple comma-separated values, Python implicitly packs those values into a tuple.)
2 - Minimal boilerplate code, perfect for rapid development.
The GOLANG Way
GoLang simplifies this process further by natively supporting multiple return values. This feature is unique and often appreciated in lightweight, high-performance applications.
Key Takeaways for GoLang:
1 - Multiple return values are a native feature.
2 - Clean and efficient, aligning with Go’s simplicity-first philosophy.
Comparison Table
Feature | Java | Python | GoLang |
Ease of Implementation | Moderate (requires wrappers) | Easy (tuples) | Very Easy (native support) |
Verbosity | High | Low | Low |
Flexibility | High | Moderate | Moderate |
Performance | Moderate | Moderate | High |
Conclusion
Each language handles the concept of returning multiple values differently, reflecting its design philosophy
Java focuses on flexibility and explicitness, often at the cost of verbosity.
Python champions simplicity and developer productivity
GoLang delivers an elegant and native approach that aligns with its efficiency-oriented nature.
Understanding these nuances can help you pick the right tool for the job and write more efficient, idiomatic code in each language.
Which language’s approach do you prefer? Let’s discuss in the comments!